Dapr JS SDK - Getting Started with PubSub Event Processing
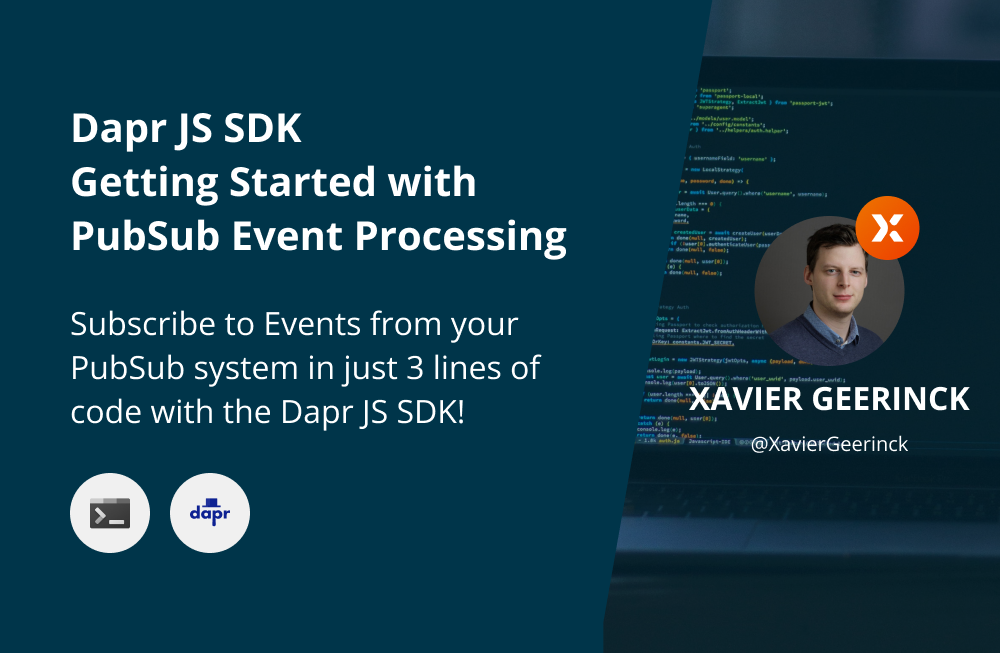
The Dapr JS SDK is created to make the life of developers easier, bringing all Dapr has to offer and wrapping it in an easy-to-use SDK. Its motto is: "Get up and running with any component in just 5 minutes"
So, let's learn how we can easily connect a PubSub component to the Dapr JS SDK and connect an Azure Event Hub instance to it in just 3 single lines of code!
Dapr provides out of the box APIs that help you build distributed applications at scale through easy-to-use building blocks such as PubSub, Bindings, Service Invocation, ... so that you don't have to write them anymore
Project Set-Up
Setting up Typescript
Refer to my previous blog post on how you set can set-up a Typescript project
Setting up the Dapr JS SDK
Installing the Dapr JS SDK is as running npm i @dapr/dapr --save
Project Layout
Now let's create the following project layout that will take care of hosting our project:
node_modules/ # Node.js modules
src/ # Source code
index.ts # Main entry file
components/ # Dapr Components
pubsub-items.yaml # Dapr PubSub component
package.json
tsconfig.json
Try it out by running npm run dev
that you configured after following the Typescript set-up post.
Configuring Package.json
Configure your package.json
and replace the scripts object with the following:
"scripts": {
"start": "ts-node --swc ./src/index.ts",
"start:dev": "nodemon --watch './src/**/*.ts' --exec 'ts-node --swc' ./src/index.ts",
"start:dapr:dev": "dapr run --app-id '$Default' --app-port 8000 --components-path ./components -- npm run start:dev"
},
This will allow us to run npm run start:dapr:dev
which does a couple of things:
- Starts our application in the Dapr Sidecar
- Monitors our application for changes and restarts when required
- Configure Dapr - Use
./components
as our components path - Configure Dapr - Use
$Default
as our application name (as required by the PubSub component as we need to name our application to the consumer group name` - Configure Dapr - Use
8000
as our app port for the server communication
Configuring pubsub-items.yaml
Now configure the component for Dapr to be able to connect to Event Hubs by creating the components/pubsub-items.yaml
file with content:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: pubsub-items
spec:
type: pubsub.azure.eventhubs
version: v1
metadata:
- name: connectionString
value: "YOUR_CONNECTION_STRING"
- name: storageAccountName
value: "YOUR_STORAGE_ACCOUNT_NAME"
- name: storageAccountKey
value: "YOUR_STORAGE_ACCOUNT_KEY"
- name: storageContainerName
value: "YOUR_CONTAINER_NAME"
Creating our application
Finally, create an application with the code below. This will take care of:
- Configuring Dapr to listen on events of Azure Event Hub
- Starting the Dapr server
import { DaprServer } from "@dapr/dapr";
async function start() {
const server = new DaprServer();
await server.pubsub.subscribe("pubsub-items", "your-entity-path-name", (data: any) => {
console.log(data);
});
await server.start();
}
start().catch(e => console.error(e));
❗Important: Use the entity path of your connection string (the Event Hub Name) as the topic name
When we run the above with npm run start:dapr
we will see an output containing our events that are being received!
Behind the Scenes
The above might all look extremely easy as we only had to write 3 single lines of code to start listening to our Event Hub Events! Behind the scenes however, the Dapr JS SDK takes care of a couple of things for you:
- Create an HTTP server: We need to be able to interface with the Dapr Sidecar. This is possible in either HTTP or gRPC and the Dapr JS SDK supports both, but it defaults to HTTP.
- Make our PubSub Subscription available to Dapr: Dapr publishes your subscription on the
/dapr/subscribe
endpoint on the HTTP Server. The JS SDK will automatically do this for you when you writeserver.pubsub.subscribe()
so you don't have to worry about how to interact with the Sidecar spec.
Summary
Hopefully by following this post you got an insight on just how easy it is to interact with the Dapr sidecar by using the JS SDK! Let us know on the official repository if you encounter any issues and how we can help you!
Member discussion