Setting up a Typescript project (Updated 2022)
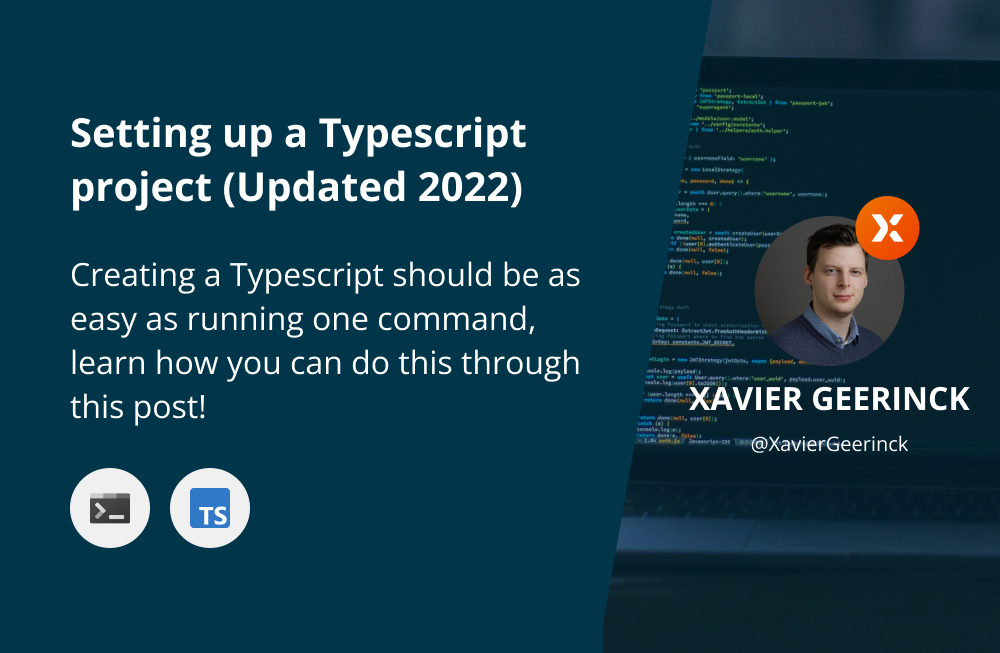
Creating a Typescript should be as easy as running one command, currently the ecosystem is moving towards it through new runtimes such as Deno or Bun or tools such as TSX but the main population sits still on the official Node.js project. Next to not having out-of-the-box Typescript support, running a Typescript project also takes a while on initial load (more on that later).
In this blog post I want to go on how you can create your own Typescript project by copy-pasting one box of commands that set it up exactly for you keeping in mind:
- Using Node.js instead of other runtimes such as deno, bun, ...
- Installing Typescript directly instead of an abstraction package
Toolchain
The toolchain I landed on is:
- Typescript: language support
ts-node
: Typescript Execution Enginenodemon
: File monitorSWC
: Transpiler for Typescript to Javascript
ts-node
will execute our Typescript project and utilize SWC
to transpile it into Javascript (which is faster than the vanilla implementation). For development we support reload
functionality by watching files with nodemon
Getting Started
Installation
Putting the above together results in the creation of our Typescript project! Just copy paste the below and run it in your terminal of choice:
npm init -y
# Install Typescript, ts-node and nodemon
pnpm i -D typescript ts-node nodemon @types/node
# Install SWC for transpiling Typescript
pnpm i -D @swc/core @swc/helpers regenerator-runtime
# Generate TSConfig and adapt for SWC usage
npx tsc --init
# Configuring tsconfig.json
# note: we specify ./dist as output dir and ./src as input
sed -i 's%// "outDir": "./"%"outDir": "./dist"%' tsconfig.json
sed -i 's%// "rootDir": "./"%"rootDir": "./src"%' tsconfig.json
# Demo Hello World
mkdir src; echo 'console.log("Hello World");' > src/index.ts
Updating Package.json
Finally, to start our application we add the start
and dev
scripts as shown below:
start
: Run the application oncedev
: Start our application in dev mode and listen to file changes, regenerating our code and running it
{
"name": "your_application",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "ts-node --swc ./src/index.ts",
"dev": "nodemon --watch './src/**/*.ts' --exec 'ts-node --swc' ./src/index.ts"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"@swc/core": "^1.2.244",
"@swc/helpers": "^0.4.11",
"nodemon": "^2.0.19",
"regenerator-runtime": "^0.13.9",
"ts-node": "^10.9.1",
"typescript": "^4.8.2"
}
}
Benchmark
Important now is to see why we are using swc
instead of the vanilla implementation, so let's quickly benchmark it!
To benchmark, we can simply run --exec 'time ts-node'
and --exec 'time ts-node --swv'
in our scripts. When executing this on a simple file with console.log("Hello World")
as content shows us on the first run:
# --exec 'time ts-node'
1.49user 0.10system 0:00.75elapsed 212%CPU (0avgtext+0avgdata 196788maxresident)k
0inputs+0outputs (1major+38672minor)pagefaults 0swaps
# --exec 'time ts-node --swc'
0.12user 0.08system 0:00.16elapsed 125%CPU (0avgtext+0avgdata 229144maxresident)k
0inputs+0outputs (0major+10503minor)pagefaults 0swaps
We thus spend 0.12s
instead of 1.49
in user space when running this command and it even uses less CPU!
Summary
Hopefully after reading this post, you are now able to get started with Typescript in just one simple copy-paste! Let me know what you think!
Member discussion