How to set up a Typescript Project with Yeoman
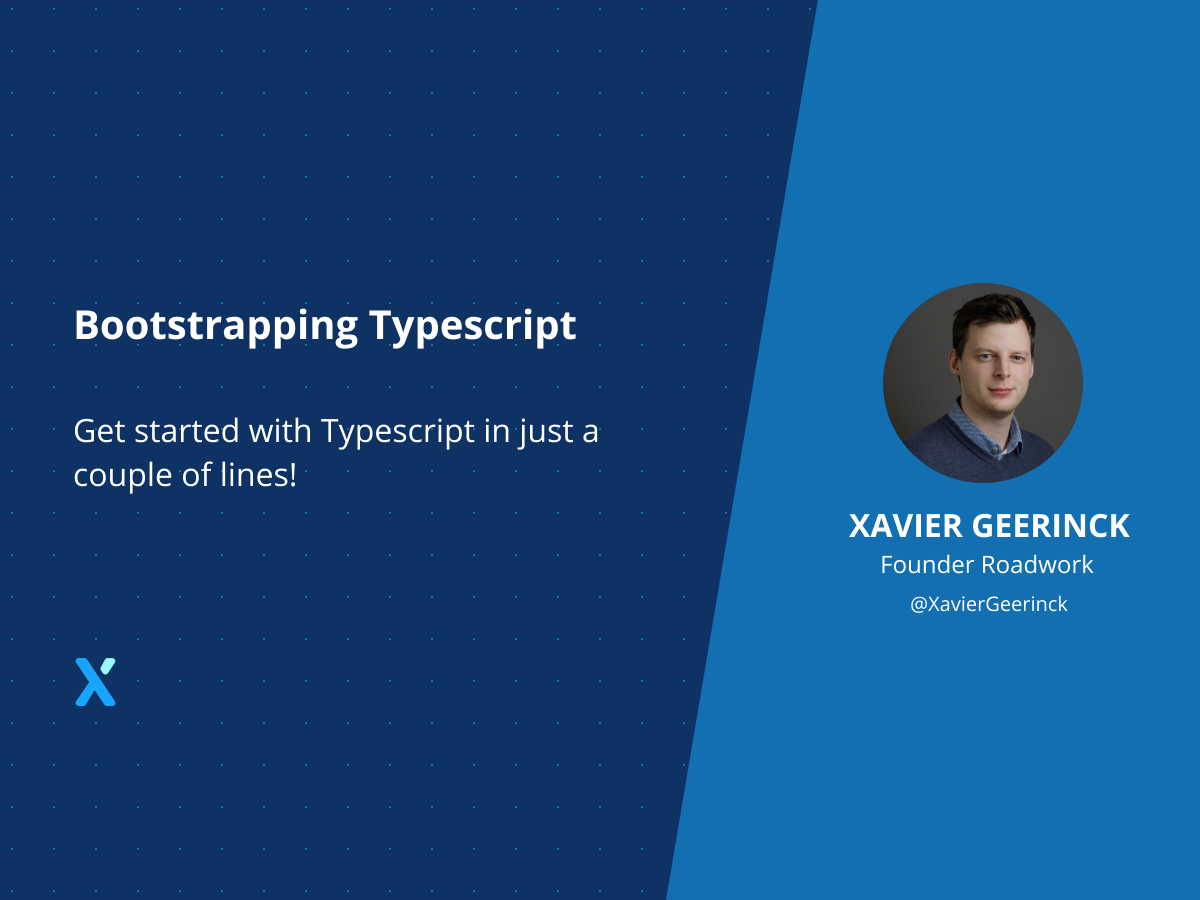
Typescript is quite cumbersome to set-up… it's not built in in Node.js but it is important to write stable code with type checking. Since I write a lot of Typescript projects, it's quite handy to have an explanation on how to set-up a typescript project quickly.
For this I start by creating a working directory for my project. E.g. my-project/ and navigate to it.
1. Installing NPM + Typescript
To set-up a Typescript project, execute the following and then following the specific steps for your Shell of choice:
# Init NPM
npm init -y
# Install Dependencies
npm install typescript ts-node nodemon rimraf --save-dev
npm install @types/node --save
./node_modules/.bin/tsc --init
# Create Base File
mkdir -p src
touch src/index.ts
# Send content to the file
cat <<EOF >> ./src/index.ts
async function main() {
console.log("Hello World")
}
main()
.catch((e) => {
console.error(e);
process.exit();
})
EOF
2. Configuring Package.json
After the project is set-up, we have to adapt our package.json
so that we are able to execute npm run start:dev
to start developing. Therefore, add the following lines to the scripts
section:
"build": "rimraf ./dist && tsc",
"start": "npm run build && node dist/index.js",
"start:dev": "npm run build && nodemon --ext \".ts,.js\" --watch \"./src\" --exec \"ts-node ./src/index.ts\""
3. Configuring tsconfig.json
Next to the package.json
we will also see a tsconfig.json
being created. In here, change the following:
"outDir": "./dist"
"rootDir": "./src"
Wich will compile our typescript project into the ./dist
folder and read the source code from the ./src
folder.
Validation
After executing the steps above, you should now be able to create code, save and have auto-reload working.
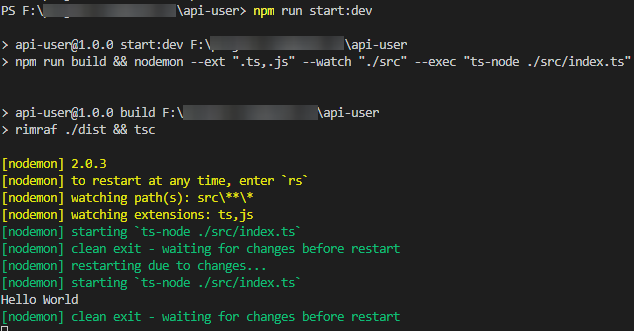
Comments ()